ASP.NET Core can serve static files—HTML files, images, JavaScript files, etc.—directly to clients. To enable ASP.NET Core to serve static files, you must use the framework’s Static Files Middleware in your application, and you must specify the necessary configuration. This article presents a discussion on how we can work with static files in ASP.NET Core.
Create an ASP.Net Core MVC project in Visual Studio
First off, let’s create an ASP.Net Core project in Visual Studio 2019. Assuming Visual Studio 2019 is installed in your system, follow the steps outlined below to create a new ASP.Net Core project in Visual Studio.
- Launch the Visual Studio IDE.
- Click on “Create new project.”
- In the “Create new project” window, select “ASP.NET Core Web Application” from the list of templates displayed.
- Click Next.
- In the “Configure your new project” window, specify the name and location for the new project. Optionally, select the “Place solution and project in the same directory” check box.
- Click Create.
- In the “Create a New ASP.NET Core Web Application” window shown next, select .NET Core as the runtime and ASP.NET Core 2.2 (or later) from the drop-down list at the top.
- Select “Web Application (Model-View-Controller)” as the project template to create a new ASP.NET Core MVC application.
- Ensure that the check boxes “Enable Docker Support” and “Configure for HTTPS” are unchecked as we won’t be using those features here.
- Ensure that Authentication is set to “No Authentication” as we won’t be using authentication either.
- Click Create.
Following these steps should result in a new ASP.NET Core MVC project ready and waiting in Visual Studio. We’ll use this project in the sections that follow to work with static files in ASP.NET Core MVC.
Install the Static Files Middleware NuGet package
Now that we have created an ASP.NET Core MVC application in Visual Studio, the next thing you should do is install the necessary NuGet package. You must add the Microsoft.AspNetCore.StaticFiles middleware in the request pipeline to enable ASP.NET Core to serve static content.
You can install this package from NuGet Package Manager inside the Visual Studio 2019 IDE. Alternatively, you can run the following command to install this package via the .NET CLI.
dotnet add package Microsoft.AspNetCore.Rewrite
Configure the Static Files Middleware in ASP.NET Core
To enable ASP.NET Core MVC to serve static files, you must configure the Static Files Middleware. The following code snippet illustrates how you can configure the Static Files Middleware in the Startup class.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { app.UseStaticFiles(); app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); endpoints.MapRazorPages(); }); }
Serve static files in ASP.NET Core
You’ll find the static files inside the wwwroot (content-root/wwwroot) folder. The static files are stored in folders named css, images, and js. All of these folders reside inside the wwwroot folder and are accessed using a relative path.
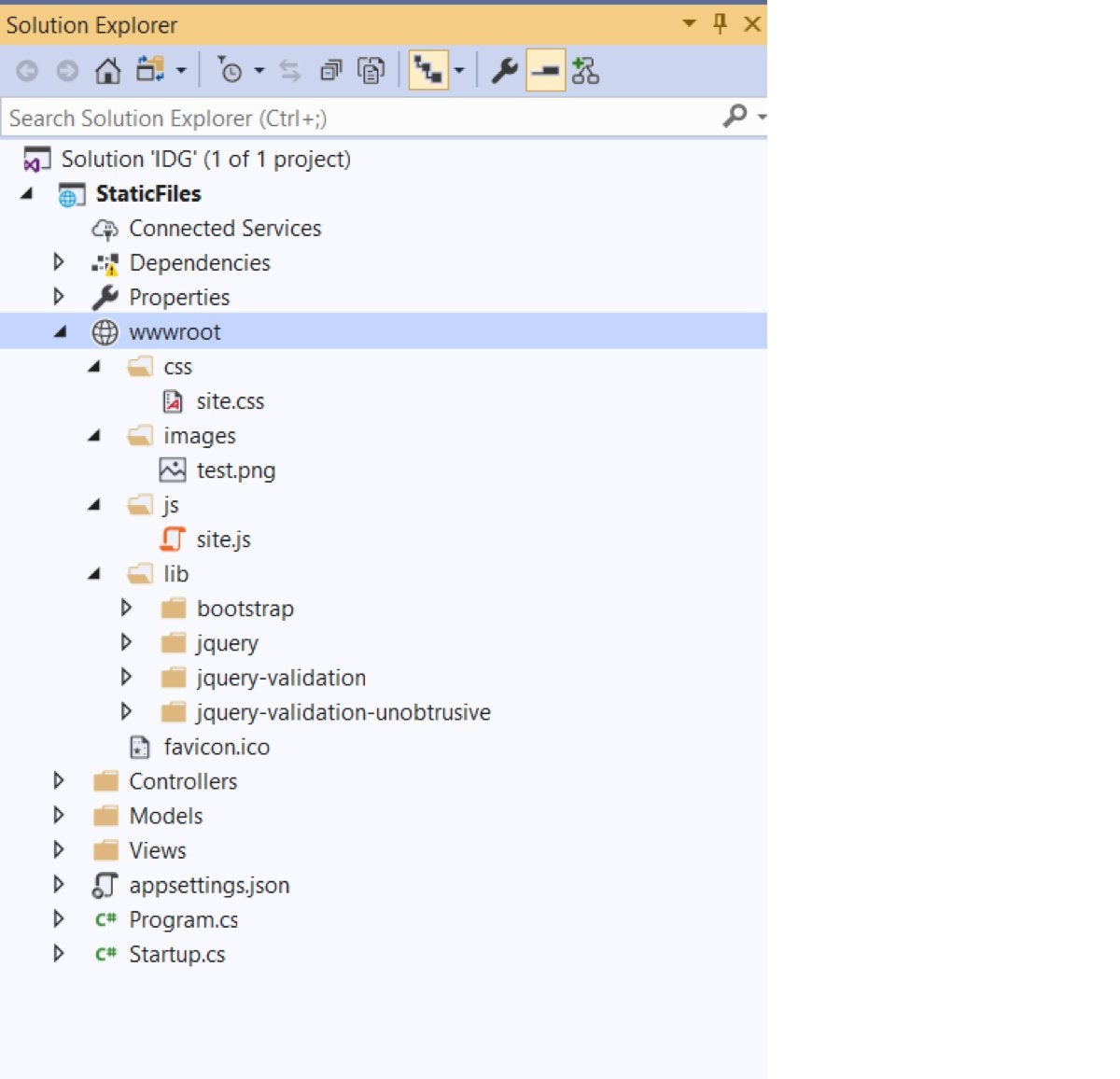
If the Static Files Middleware has already been installed and configured in your project, you can use the following URL to access an image named test.png:
http://localhost:44399/images/test.png
When you execute the application, you can see the image displayed in the web browser. Note the URL in the web browser.
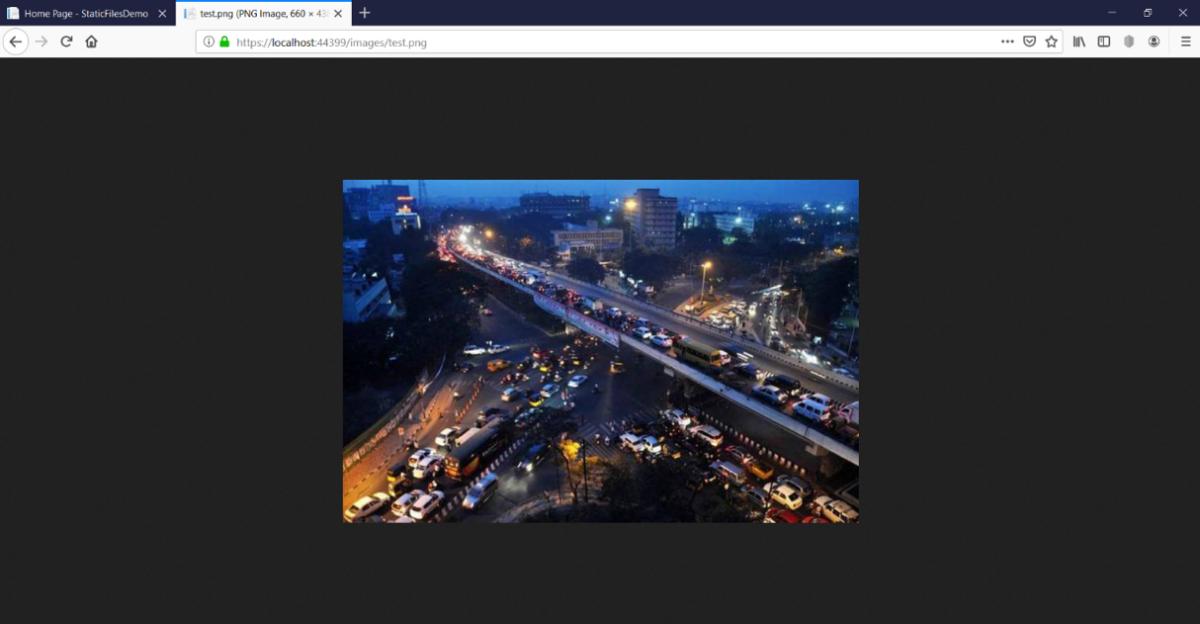
IDG
Now imagine that you’ll have to serve static files that reside outside of the wwwroot folder. Assume that there is a folder named IDGStaticFiles that contains the test.png file. The following code snippet illustrates how you can configure the Static Files Middleware to serve files that reside outside of the wwwroot folder.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory) { app.UseStaticFiles(); app.UseStaticFiles(new StaticFileOptions() { FileProvider = new PhysicalFileProvider( Path.Combine(Directory.GetCurrentDirectory(), @"IDGStaticFiles")), RequestPath = new PathString("/StaticFiles") }); }
Enable directory browsing in ASP.NET Core
Directory browsing is a feature in web applications that allows users to browse the files and directories inside a specified directory. Note that for security reasons, this feature is disabled by default. You can enable directory browsing in an ASP.NET Core application by using the UseDirectoryBrowser extension method in the Configure method of the Startup class.
The following code snippet illustrates how you can add the services required to enable directory browsing to the request processing pipeline.
public void ConfigureServices(IServiceCollection services) { services.AddDirectoryBrowser(); }
Next, write the following code in the Configure method of the Startup class to use this middleware.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { app.UseStaticFiles(); app.UseStaticFiles(new StaticFileOptions() { FileProvider = new PhysicalFileProvider( Path.Combine(Directory.GetCurrentDirectory(), @"wwwroot")), RequestPath = new PathString("/IDGImages") }); app.UseDirectoryBrowser(new DirectoryBrowserOptions() { FileProvider = new PhysicalFileProvider( Path.Combine(Directory.GetCurrentDirectory(), @"wwwroot\images")), RequestPath = new PathString("/IDGImages") }); }
When you run the application and specify IDGImages in the path string, you’ll be able to see the contents of the wwwroot\images folder.
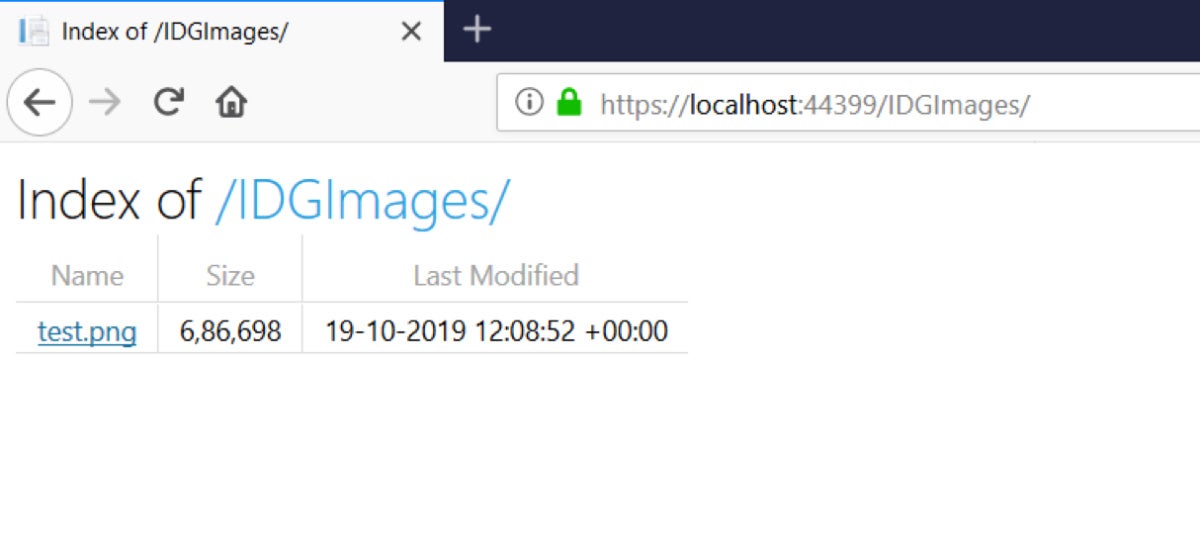
IDG
Secure file access in ASP.NET Core
Since there are no authorization checks by default, how can you secure access to the files that are served by ASP.NET Core? Note that the files that are present in the wwwroot folder are publicly available. To secure these files, it is imperative that you move these files to a different folder, i.e., to a folder that is outside the wwwroot folder. Lastly, to serve these files you can leverage an action method in your controller class that returns a FileResult instance.
Serve a default page in ASP.NET Core
Finally, you can take advantage of the UseDefaultFiles extension method to serve a default page. Here’s how you can configure this extension method in the Startup class.
public void Configure(IApplicationBuilder app) { app.UseDefaultFiles(); app.UseStaticFiles(); }
The UseFileServer extension method combines the capabilities of the UseStaticFiles, UseDefaultFiles, and UseDirectoryBrowser extension methods. If you would like to leverage all three capabilities using the UseFileServer extension method, you can write the following code in the Configure method of the Startup class.
app.UseFileServer(enableDirectoryBrowsing: true);